LLD basics— [Notes]
2 min readNov 26, 2022
[Refer LLD Index for all LLD topics]
· Sequential vs Procedural languages
· OOP | Procedural vs Object Oriented languages
∘ Method vs Functional Procedure
∘ Fields + Methods?
∘ State
∘ Polymorphism
· TODO
Sequential vs Procedural languages
- Sequential languages executes code line by line
- Procedural languages are Sequential languages that support procedures/functions
OOP | Procedural vs Object Oriented languages
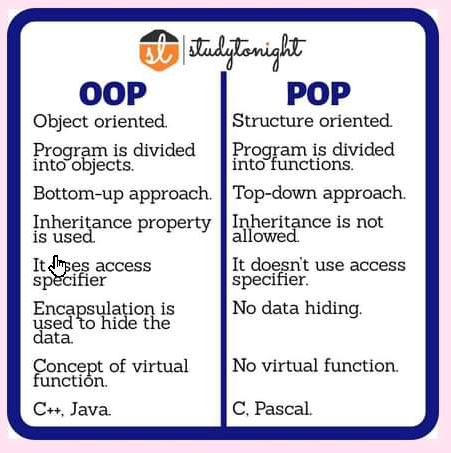
//Procedural
int main(){
String animal_name = "Zebra";
int animal_id = 123;
String animal_sound = "bow"
System.out.println(animal_name);
System.out.println(animal_id);
System.out.println(animal_sound);
}
Class : represents a real work entity.
//OOP
class Animal {
String name; // fields/attributes/properties
int id;
String sound;
public Animal(String name, int id, String sound){
this.name = name;
this.id = id;
this.sound = sound;
}
public String getName(){ // behaviours
return this.sound;
}
}
int main(){
Animal zerba = new Animal("zebra", 123, "bow"); //object
}
Method vs Functional Procedure
- Method is basically the function in the context of a class
- for java [object oriented language]— methods and procedure can be same.
Fields + Methods?
- Fields + Methods are known as Members of a class
State
- The value of every field of a class at a particular time is known as the state of the class
Favor interfaces >> Inheritance
Polymorphism
- When we can assign an object of child class to parent class
TODO
- why web apps does not have main method?
- Procedural vs Functional languages
- Object Oriented vs Functional languages